Se termino el modo computadora vs humano
parents
Showing
README.MD
0 → 100644
css/styles.css
0 → 100644
img/O.png
0 → 100644
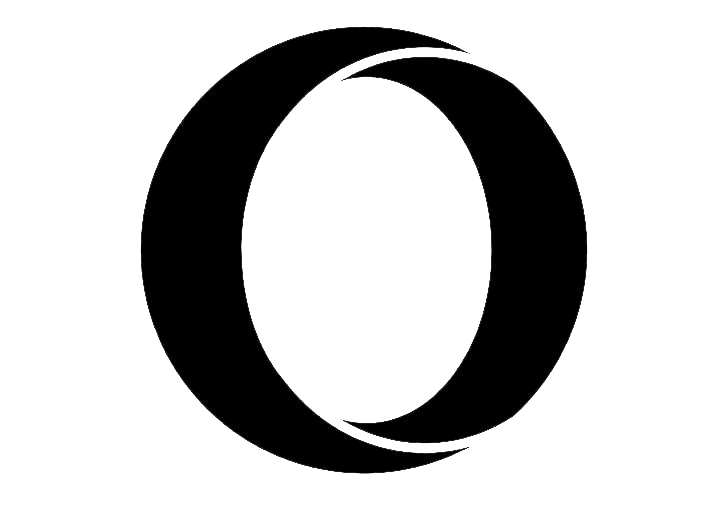
21.4 KB
img/x.png
0 → 100644
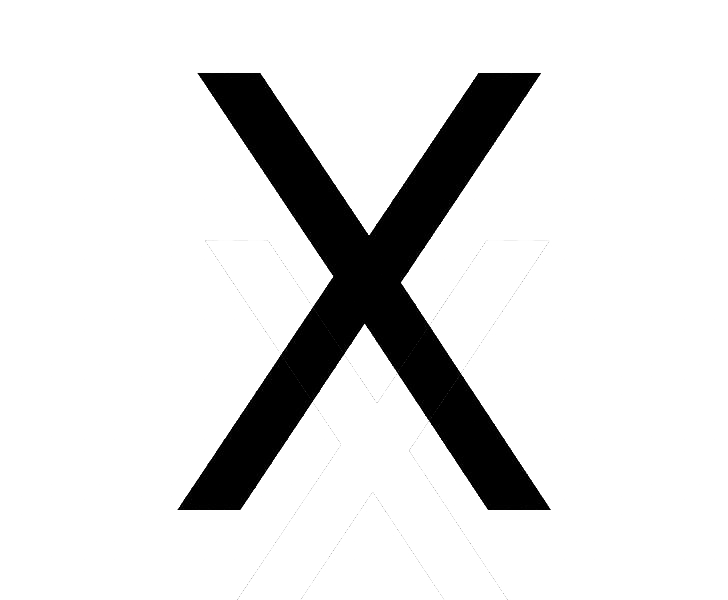
33.2 KB
js/main.js
0 → 100644
tateti.html
0 → 100644
Please
register
or
sign in
to comment