primer commit en Roshka
parents
Showing
dia1/toro_vaca.py
0 → 100644
dia2/Bisiesto.java
0 → 100644
dia2/Box.java
0 → 100644
dia2/ClassPila.java
0 → 100644
dia2/Estaciones.java
0 → 100644
dia2/Lenguajes.java
0 → 100644
dia2/estaciones.c
0 → 100644
dia3/ClaseNotas.java
0 → 100644
dia3/Herencia.java
0 → 100644
dia3/Operaciones.java
0 → 100644
dia3/PruebaStatic.java
0 → 100644
dia3/SortPila.java
0 → 100644
dia3/dvdrental.sql
0 → 100644
dia3/dvdrental.tar
0 → 100644
File added
dia3/entidad_relacion.png
0 → 100644
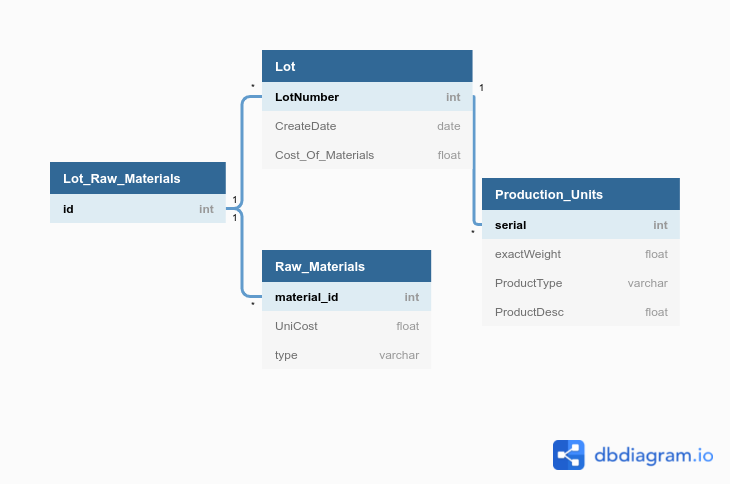
37.8 KB
dia3/polimorfismo.java
0 → 100644
dia3/ups.sql
0 → 100644
dia3/usp_entidad_relacion.png
0 → 100644
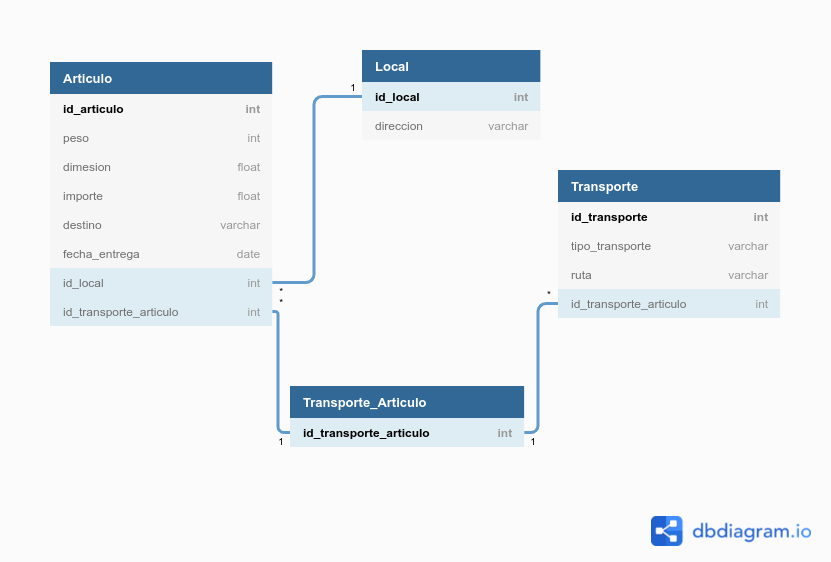
44.6 KB
dia4/InterfacesDia3.java
0 → 100644
dia4/SelectAndWhereDia3.sql
0 → 100644
dia4/calculadora.html
0 → 100644
dia5/Twitter.java
0 → 100644
dvdrental.tar
0 → 100644
File added
dvdrental.zip
0 → 100644
File added
dvdrental/3055.dat
0 → 100644
This diff is collapsed.
Click to expand it.
dvdrental/3057.dat
0 → 100644
dvdrental/3059.dat
0 → 100644
dvdrental/3061.dat
0 → 100644
This source diff could not be displayed because it is too large.
You can
view the blob
instead.
dvdrental/3062.dat
0 → 100644
This source diff could not be displayed because it is too large.
You can
view the blob
instead.
dvdrental/3063.dat
0 → 100644
This diff is collapsed.
Click to expand it.
dvdrental/3065.dat
0 → 100644
This diff is collapsed.
Click to expand it.
dvdrental/3067.dat
0 → 100644
This diff is collapsed.
Click to expand it.
dvdrental/3069.dat
0 → 100644
dvdrental/3071.dat
0 → 100644
This source diff could not be displayed because it is too large.
You can
view the blob
instead.
dvdrental/3073.dat
0 → 100644
dvdrental/3075.dat
0 → 100644
This source diff could not be displayed because it is too large.
You can
view the blob
instead.
dvdrental/3077.dat
0 → 100644
This source diff could not be displayed because it is too large.
You can
view the blob
instead.
dvdrental/3079.dat
0 → 100644
dvdrental/3081.dat
0 → 100644
dvdrental/restore.sql
0 → 100644
This diff is collapsed.
Click to expand it.
dvdrental/toc.dat
0 → 100644
File added
Please
register
or
sign in
to comment