Initial commit
parents
Showing
Dia 1/Torosyvacas.java
0 → 100644
Dia 2/Lot.png
0 → 100644
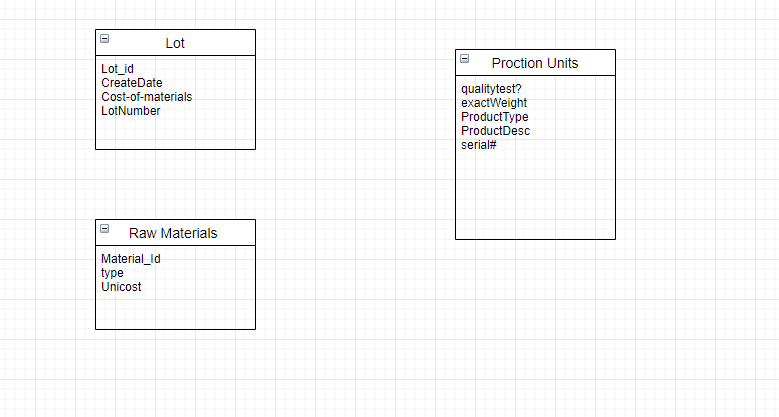
8.46 KB
Dia 2/UML.png
0 → 100644
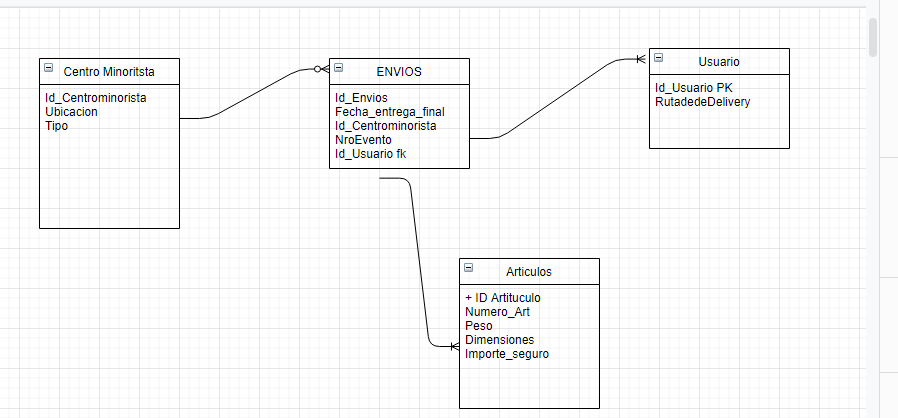
30.5 KB
Dia 2/switch_meses.java
0 → 100644
Dia 3/ConsultasFilm.docx
0 → 100644
File added
Dia 3/Dia3.docx
0 → 100644
File added
Dia 3/Mochila.java
0 → 100644
Dia 3/Pila.java
0 → 100644
Dia 3/~$Dia3.docx
0 → 100644
File added
Dia 3/~$nsultasFilm.docx
0 → 100644
File added
Dia 4/Calculadora.html
0 → 100644
Dia 4/Select Where.docx
0 → 100644
File added
Dia 4/estilo.css
0 → 100644
Dia 4/funcionalidad.js
0 → 100644
Please
register
or
sign in
to comment