JavaCleta 1.0
parents
Showing
Readme.md
0 → 100644
java-e006/_classpath.xml
0 → 100644
java-e006/_project.xml
0 → 100644
java-e006/bin/Bicicleta.class
0 → 100644
File added
java-e006/bin/Something$1.class
0 → 100644
File added
java-e006/bin/Something$2.class
0 → 100644
File added
java-e006/bin/Something$3.class
0 → 100644
File added
java-e006/bin/Something.class
0 → 100644
File added
java-e006/bin/bici.png
0 → 100644
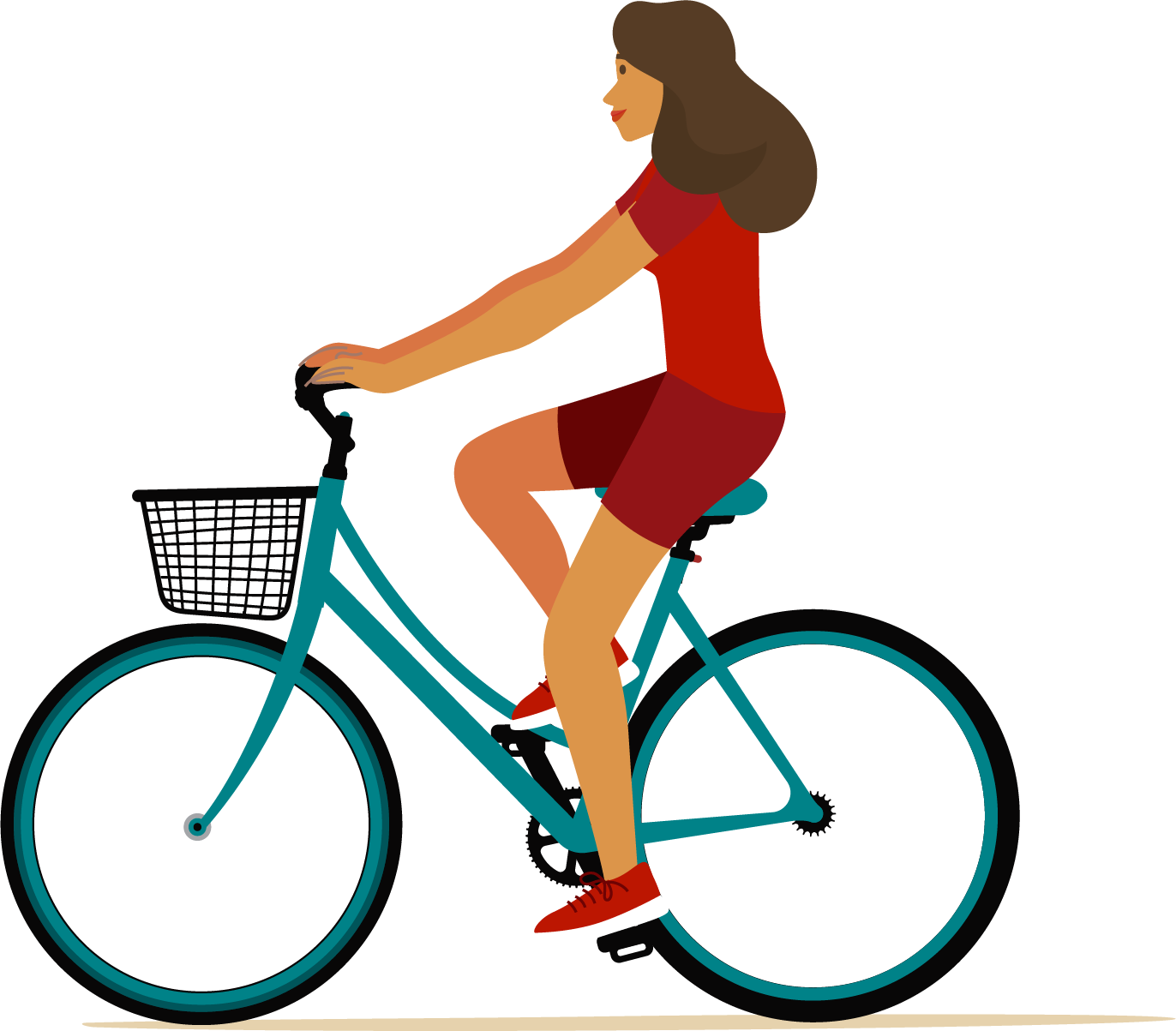
162 KB
java-e006/bin/bici2.png
0 → 100644
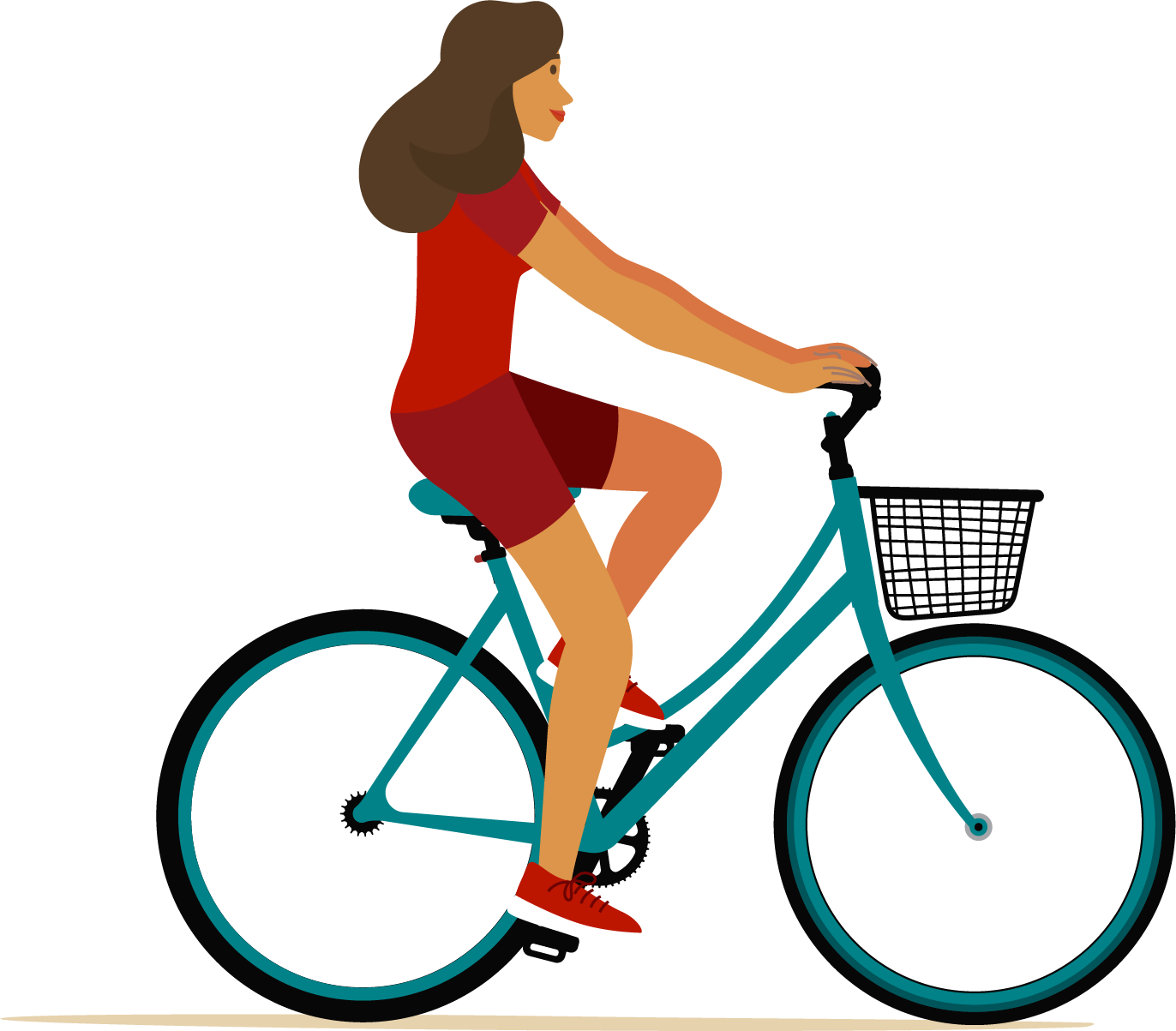
164 KB
java-e006/bin/fondo.png
0 → 100644
This diff is collapsed.
Click to expand it.
java-e006/src/Bicicleta.java
0 → 100644
java-e006/src/Something.java
0 → 100644
Please
register
or
sign in
to comment